How to Read a Key From Web.config
Configuration direction is something we cannot do without. It is a must-have feature. In this blog mail service, I am going to discuss how to manage and access configuration in ASP.Net Core applications. I will use ASP.Net Core 3.i for demonstrating the example here.
Configuration direction in ASP.Net Core has improved significantly compared to how information technology was managed in the legacy .Net Framework.
Firstly, it is saved every bit JSON instead of XML compared to the legacy .Internet Framework. This makes reading and managing the configuration file extremely simple and user friendly.
Creating a new ASP.Net Cadre Awarding
To first this example, first of all, I will create a new ASP.NET Cadre 3.1 Web API Application.
Firstly, to exercise that, I volition open up Visual Studio 2019. Once Visual Studio opens up, I will select the menuFile -> New -> Projection. This will open up theCreate a new Project project popup window.
Secondly, in theCreate a new Project popup window, I will select ASP.Internet Core Spider web Awarding from the projection template and click on theNext push button.
Thirdly, on the next page, I will provide the name of the application asConfig.Demo
and click on theCreate button.
Finally, on the final page, I will select theAPI template option. And I volition keep other values default (ASP.Cyberspace Core 3.one) and click on theCreate button.
The configuration file
Past default, the Visual Studio project template will create an appsettings.json
and appsettings.Evolution.json
file when a new projection is created.
These are the files where nosotros salve configurations based on the environs, where appsettings.json
is the default configuration file. The surround name is normally available as an environment variable, with key "ASPNETCORE_ENVIRONMENT".
CreateDefaultBuilder
using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Hosting; namespace Config.Demo { public static grade Plan { public static void Chief(cord[] args) { CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>(); }); } }
Inside of the Program
course, within of the CreateHostBuilder
function, we make a phone call to Host.CreateDefaultBuilder
. If we peek into the definition of the CreateDefaultBuilder
you can encounter information technology does the following:
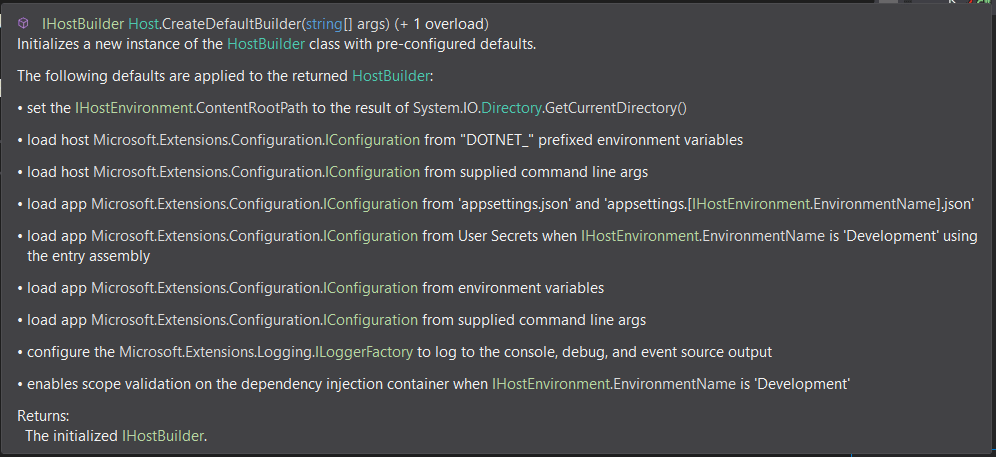
- Firstly, It sets the
ContentRootPath
of theIHostEnvironment
to the current directory - Secondly, Information technology goes and figures out the
appsettings.json
configuration file - And then also information technology loads the
appsettings.{surroundings}.json
config - Thirdly, it goes and information technology loads environment variables
- Fourthly, it loads anything passed to the arguments
Accessing configurations from Appsettings
For reading configurations from appsettings.evolution.json
, firstly, let united states of america add together a couple of entries to the appsetting.development.json
.
Now, for the first entry, I will add together a key Version
, which volition take a decimal value associated with it.
Adjacent, for the second entry, I will add a key Settings
, which volition have a complex object associated with it.
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft": "Alert", "Microsoft.Hosting.Lifetime": "Information" } }, "Version": "1.0", "Settings": { "Database": "SQL" } }
Now we will go to the Startup
class, and try to access these two configuration items from the ConfigureServices
method and print to the console as output.
To do that, I volition admission these values within of the ConfigureServices
method of the Startup
class. And at that place are two ways to access the values from the configuration file.
- Firstly using
Configuration.GetValue
- Secondly using the indexer on
Configuration
belongings
using Arrangement; using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace Config.Demo { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { go; } // This method gets called by the runtime. Utilize this method to add services to the container. public void ConfigureServices(IServiceCollection services) { services.AddControllers(); var version = Configuration.GetValue<string>("Version"); var db = Configuration["Settings:Database"]; Console.WriteLine(version); Console.WriteLine(db); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } } }
At present if I run the application, I volition see the Version
and Database
are printed in the console.
And equally you can run across from the code, we can access multiple layers of configuration values by separating the keys with :
, for example, hither we are using Settings:Database
to admission the value of Database
which is at the adjacent level of Settings
.
Accessing configurations from Controller
At present that nosotros are able to access configuration within of the Startup
class, we can add the configurations to the dependency injection container and admission it from the controller.
To practice that I will update the WeatherForecastController
class and inject cord version
to the constructor. And I will update the implementation of the Get
method to return this version
.
using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Logging; namespace Config.Demo.Controllers { [ApiController] [Route("[controller]")] public class WeatherForecastController : ControllerBase { private readonly ILogger<WeatherForecastController> _logger; private readonly string version; public WeatherForecastController( ILogger<WeatherForecastController> logger, cord version) { _logger = logger; this.version = version; } [HttpGet] public cord Get() { return version; } } }
In one case the controller is fix, I volition go to the Startup
course to add the Version
configuration to the dependency injection. To do that nosotros tin can write the post-obit code in the ConfigureServices
method:
services.AddSingleton(version);
This will add together the Version
as a sting to the dependency injection container.
using System; using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace Config.Demo { public form Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } // This method gets chosen by the runtime. Utilise this method to add together services to the container. public void ConfigureServices(IServiceCollection services) { services.AddControllers(); var version = Configuration.GetValue<string>("Version"); var db = Configuration["Settings:Database"]; services.AddSingleton(version); Console.WriteLine(version); Console.WriteLine(db); } // This method gets called past the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } } }
At present, if I run the application and admission /weatherforecast, I volition see that version ane.0 is returned to the output.
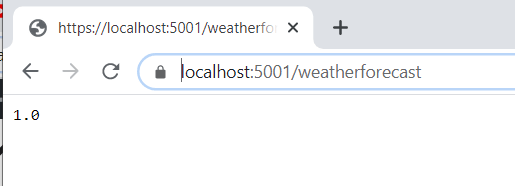
Admission complex configuration object
Now allow usa add together another configuration section in the asspettings.evolution.json
configuration file. And I will name this configuration equally Custom
, and it volition exist a multilevel configuration item. And Custom
have ConfigValue
as child level configuration.
{ "Logging": { "LogLevel": { "Default": "Information", "Microsoft": "Alarm", "Microsoft.Hosting.Lifetime": "Data" } }, "Version": "1.0", "Settings": { "Database": "SQL" }, "Custom": { "ConfigValue": "Custom configuration" } }
Once the configuration detail is added, firstly, I will create a class called Custom
. And within the grade, I volition have a single property ConfigValue
of type string.
namespace Config.Demo { public class Custom { public string ConfigValue { get; fix; } } }
Secondly, I will become to the Startup
form and add this configuration value to the dependency injection container. To do that, I will use the Bind
function of the IConfigurationSection
instance, which is returned from the Configuration.GetSection
method.
var custom = new Custom(); Configuration.GetSection("Custom").Bind(custom);
Thirdly, I can add this Custom object to the dependency injection container using services.AddSingleton
method.
using System; using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace Config.Demo { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { go; } // This method gets called by the runtime. Use this method to add services to the container. public void ConfigureServices(IServiceCollection services) { services.AddControllers(); var version = Configuration.GetValue<string>("Version"); var db = Configuration["Settings:Database"]; services.AddSingleton(version); var custom = new Custom(); Configuration.GetSection("Custom").Bind(custom); services.AddSingleton(custom); Panel.WriteLine(version); Console.WriteLine(db); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } } }
Fourthly, I will become to the WeatherForecastController
class and inject the Custom
object to the controller. And so I volition update the Get
method to return the value of ConfigValue
property of the Custom
class as output.
using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Logging; namespace Config.Demo.Controllers { [ApiController] [Route("[controller]")] public grade WeatherForecastController : ControllerBase { private readonly ILogger<WeatherForecastController> _logger; private readonly string version; individual readonly Custom custom; public WeatherForecastController( ILogger<WeatherForecastController> logger, string version, Custom custom) { _logger = logger; this.version = version; this.custom = custom; } [HttpGet] public string Get() { return custom.ConfigValue; } } }
Finally, if I run the awarding, I volition see the value of ConfigValue
, which is "Custom configuration" returned from the /weatherforecast API.
The more simplified way to add together configuration value to the dependency injection container is to use the Configure
method of the IServiceCollection
method, instead of using the Bind
characteristic. But in that case, the dependency injection container will inject a generic IOptions
object for the type.
To demonstrate that, firstly, I will update the implementation of the Startup
class'southward ConfigureServices
method to utilise the following lawmaking to configure the Custom
configuration section to the dependency injection container.
services.Configure<Custom>(Configuration.GetSection("Custom"));
Updated lawmaking for the Startup
class is as follows:
using System; using Microsoft.AspNetCore.Architect; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace Config.Demo { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } // This method gets called by the runtime. Apply this method to add together services to the container. public void ConfigureServices(IServiceCollection services) { services.AddControllers(); var version = Configuration.GetValue<cord>("Version"); var db = Configuration["Settings:Database"]; services.AddSingleton(version); services.Configure<Custom>(Configuration.GetSection("Custom")); Panel.WriteLine(version); Console.WriteLine(db); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } } }
Secondly, I will change the implementation of the WeatherForecastController
course, to have IOptions
of Custom
instead of just Custom
class as a dependency.
using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Logging; using Microsoft.Extensions.Options; namespace Config.Demo.Controllers { [ApiController] [Route("[controller]")] public class WeatherForecastController : ControllerBase { private readonly ILogger<WeatherForecastController> _logger; private readonly string version; private readonly Custom custom; public WeatherForecastController( ILogger<WeatherForecastController> logger, cord version, IOptions<Custom> custom) { _logger = logger; this.version = version; this.custom = custom.Value; } [HttpGet] public cord Go() { render custom.ConfigValue; } } }
At present, if I run the awarding, I volition run across the exact same output every bit before.
Accessing Environment variables
This is one feature that I absolutely love well-nigh .Internet Core, is that we tin can access even environment variables through the same IConfiguration
object. Which is really helpful.
To demonstrate this, firstly, I will add together a new environment variable named TEST_ENV in the environment variables, and I will set its value to "Test_Environment_Variable".
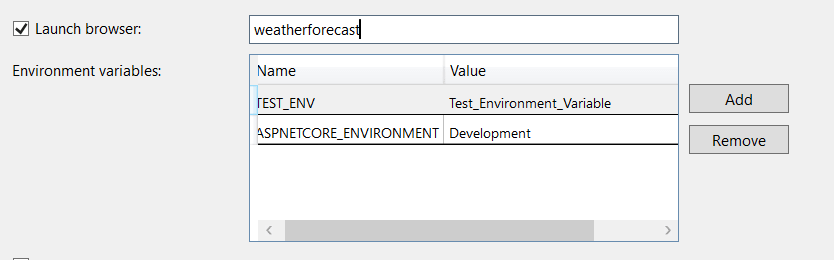
Secondly, I volition go to the Startup
class and admission this surround variable using Configuration["TEST_ENV"]
and impress it out to the console.
using Organisation; using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace Config.Demo { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { go; } // This method gets called by the runtime. Apply this method to add services to the container. public void ConfigureServices(IServiceCollection services) { services.AddControllers(); var version = Configuration.GetValue<cord>("Version"); var db = Configuration["Settings:Database"]; services.AddSingleton(version); services.Configure<Custom>(Configuration.GetSection("Custom")); Panel.WriteLine(version); Panel.WriteLine(db); Console.WriteLine(Configuration["TEST_ENV"]); } // This method gets chosen by the runtime. Utilise this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseHttpsRedirection(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } } }
At present if I run the application, I can encounter the environs variable output in the console.
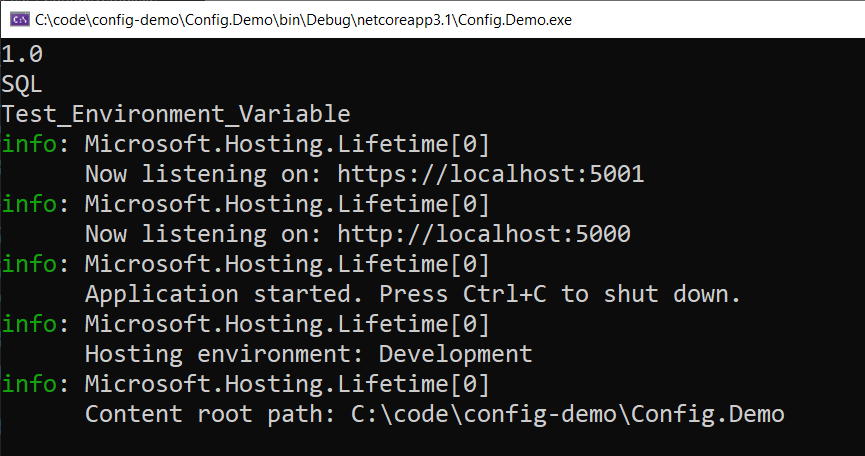
Accessing Custom Configuration File
We tin can too add together custom JSON configuration files to our projects and access them using the IConfiguration
object. To demonstrate that, firstly, permit us add a new configuration file named CustomConfig.json
to the projection. And this configuration file volition have a single configuration section CustomConfig
.
{ "CustomConfig": "Added custom config" }
Secondly, nosotros will update the Programme
class's CreateHostBuilder
method to add the extension method ConfigureAppConfiguration
. And to the IConfigurationBuilder
delegate, we will call the AddJsonFile
extension method to add the custom configuration file.
using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.Hosting; namespace Config.Demo { public static course Plan { public static void Main(cord[] args) { CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>() .ConfigureAppConfiguration(c => c.AddJsonFile("CustomConfig.json")); }); } }
At present I volition get and update the Startup
grade's ConfigureServices
method to access the newly added custom configuration value.
Console.WriteLine(Configuration["CustomConfig"]);
Now if I run the awarding, I will run across the value of CustomConfig
node is printed in the console output.
Overriding configuration with arguments
We can update the configurations from the arguments passed to the application. To demonstrate this, permit us say, we want to override the Version
number of the appsettings.development.config
from the arguments passed to the application.
Nosotros can prepare up the arguments in the Projection Properties window in the Debug tab equally shown beneath.

One time nosotros practise that, nosotros can update the CreateHostBuilder
method of Program
class to replace the configuration value from the arguments passed.
To exercise that firstly, I will create a new Dictionary
example, where I volition create a mapping of the argument keys with the configuration keys.
var replacement = new Lexicon<string, string> { {"-v", "Version" } };
Secondly, I will call the AddCommandLine
extension method on the IConfigurationBuilder
instance to replace the configurations with control line arguments.
using System.Collections.Generic; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.Hosting; namespace Config.Demo { public static class Program { public static void Main(string[] args) { CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureWebHostDefaults(webBuilder => { var replacement = new Lexicon<string, string> { {"-5", "Version" } }; webBuilder.UseStartup<Startup>() .ConfigureAppConfiguration(c => c.AddJsonFile("CustomConfig.json") .AddCommandLine(args, replacement)); }); } }
Now if nosotros run our application, we will see that the value of Version
in the console output from the Startup
class where we are accessing it through Configuration.GetValue
will print out as 2.0 now.
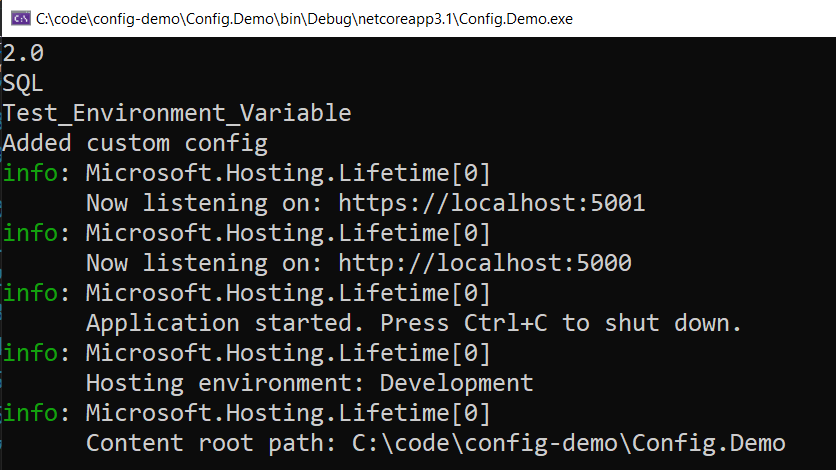
Conclusion
As y'all can see, configuration management in ASP.NET Core is extremely streamlined which makes using configurations a cakewalk in ASP.Cyberspace Core applications.
I have captured the entire process in the YouTube video here.
The source code for this blog post is available in my GitHub repo here.
Source: https://dotnetcorecentral.com/blog/configuration-in-asp-net-core/
0 Response to "How to Read a Key From Web.config"
Post a Comment